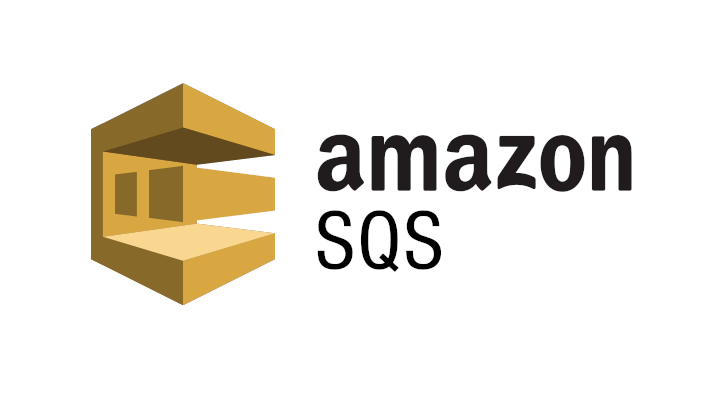
Understanding AWS SQS (Simple Queue Service)
AWS SQS (Simple Queue Service) is a fully managed message queuing service that allows you to decouple and scale microservices, distributed systems, and serverless applications. It enables reliable communication between different parts of an application, even if they are running at different speeds or are temporarily unavailable.
In this guide, we’ll explore what AWS SQS is, how it works, its history, and provide an example of implementing it with Python.
What is AWS SQS?
AWS SQS is a message queuing service provided by Amazon Web Services (AWS). It allows you to send, store, and receive messages between software components at any volume. SQS makes it easy to build distributed, decoupled applications by ensuring that components can communicate asynchronously.
Message queues, like SQS, help ensure that messages are reliably transmitted between different services. If one service is temporarily down, the message will stay in the queue until the service becomes available again. This allows for more resilient and scalable architectures.
With SQS, messages are stored in a queue, and other systems or services can retrieve these messages at their own pace, allowing them to process the messages in the order they are received. This is especially useful in decoupled or microservices architectures where different parts of the system need to communicate but operate independently.
How Does AWS SQS Work?
AWS SQS is designed to handle high throughput and ensures that messages are delivered once and only once (although sometimes they might be delivered more than once in certain cases, so your application should be idempotent to handle that). The key components of SQS are:
- Queue: The storage for messages. You can create two types of queues in SQS: Standard Queues and FIFO (First-In-First-Out) Queues.
- Messages: Data or information that is sent through the queue. These messages can be of any data type (text, JSON, etc.).
- SendMessage: API operation to send a message to the queue.
- ReceiveMessage: API operation to receive a message from the queue. A consumer will pull messages from the queue.
- DeleteMessage: API operation to delete a message from the queue once it’s processed successfully.
SQS provides a simple interface for sending and receiving messages asynchronously. Each message is stored in the queue until the consumer retrieves it, processes it, and deletes it.
Types of SQS Queues
AWS offers two types of SQS queues:
- Standard Queue: These queues offer at least once delivery and best-effort ordering. Standard queues are highly scalable and can handle nearly unlimited throughput, making them ideal for most use cases.
- FIFO Queue: FIFO queues provide exactly-once message delivery and ensure that messages are processed in the exact order that they are sent. FIFO queues are ideal when the order of message processing is important.
Why Use AWS SQS?
Here are some key benefits of using AWS SQS:
- Decoupling of services: SQS allows different components of a system to work independently of each other. One component can send a message to the queue without worrying about the state or availability of the other component.
- Scalability: SQS can handle an enormous number of messages and automatically scales to accommodate demand, making it ideal for high-volume applications.
- Fault tolerance: SQS ensures that messages are reliably delivered and can be processed even in cases of temporary service failure.
- Simple to use: AWS SQS is a fully managed service, meaning AWS takes care of the infrastructure, scaling, and availability, so you don’t need to worry about managing the queue servers.
History of Message Queuing Services
Before the advent of cloud-based solutions like AWS SQS, message queuing systems were a fundamental part of traditional IT infrastructure. They were commonly used to manage communication between different components of an enterprise application, particularly in environments where services needed to work asynchronously or at different speeds. Some popular message queuing systems that existed prior to SQS include:
- IBM MQ (formerly MQSeries): One of the earliest and most widely used enterprise messaging systems, IBM MQ has been in operation since the 1990s. It provides reliable message delivery, integration between disparate applications, and is commonly used for large enterprise systems where message-driven communication is necessary.
- RabbitMQ: An open-source message broker originally developed by VMware. It uses the AMQP (Advanced Message Queuing Protocol) and has been popular in the developer community for building scalable, fault-tolerant applications. RabbitMQ has been widely adopted in both on-premises and cloud environments for managing queues and message flows.
- Apache Kafka: Originally developed by LinkedIn and now an Apache project, Kafka is a distributed event streaming platform often used for handling real-time data feeds. While Kafka is more focused on high-throughput event streaming, it is also used to implement message queuing systems due to its ability to store large volumes of messages efficiently.
- ActiveMQ: Another open-source messaging system developed by Apache Software Foundation, ActiveMQ supports a variety of messaging protocols, including JMS (Java Message Service). It is widely used in applications where distributed messaging is necessary for scalability.
These systems were typically hosted on physical or virtual machines, requiring significant setup, configuration, and maintenance. As a result, using traditional message queuing systems often came with operational complexity and high overhead costs in terms of infrastructure management, scaling, and fault tolerance.
AWS SQS, on the other hand, offers all the benefits of these traditional message queuing systems but with the added advantages of being fully managed, highly scalable, and easily integrated into cloud-native architectures. By removing the need for infrastructure management and scaling, AWS SQS makes it easier for developers to focus on building and deploying applications without worrying about underlying message queue infrastructure.
Implementing AWS SQS in Python
To interact with SQS using Python, you can use the boto3
library, which is the AWS SDK for Python. Below is an example of how to create an SQS queue, send a message to it, and then receive and delete that message.
Step 1: Install boto3
First, make sure you have boto3
installed:
pip install boto3
Step 2: Create a Queue
To create a queue, you can use the create_queue
method provided by boto3
. In this example, we'll create a standard SQS queue:
import boto3
# Initialize SQS client
sqs = boto3.client('sqs')
# Create a new SQS queue
response = sqs.create_queue(
QueueName='MyQueue'
)
# Print the URL of the created queue
print(f'Queue URL: {response["QueueUrl"]}')
Step 3: Send a Message to the Queue
Next, we will send a message to the queue we just created using the send_message
method:
# Send a message to the SQS queue
response = sqs.send_message(
QueueUrl='https://sqs.us-east-1.amazonaws.com/123456789012/MyQueue', # Replace with your Queue URL
MessageBody='Hello, this is a test message'
)
# Print the message ID of the sent message
print(f'Message ID: {response["MessageId"]}')
Step 4: Receive and Process the Message
Once the message is sent, we can receive and process it using the receive_message
method:
# Receive a message from the queue
response = sqs.receive_message(
QueueUrl='https://sqs.us-east-1.amazonaws.com/123456789012/MyQueue', # Replace with your Queue URL
MaxNumberOfMessages=1,
WaitTimeSeconds=10 # Long poll for up to 10 seconds
)
# Check if messages are available
if 'Messages' in response:
message = response['Messages'][0]
print(f'Received message: {message["Body"]}')
# Delete the message from the queue after processing
sqs.delete_message(
QueueUrl='https://sqs.us-east-1.amazonaws.com/123456789012/MyQueue', # Replace with your Queue URL
ReceiptHandle=message['ReceiptHandle']
)
print('Message deleted from queue')
else:
print('No messages available')
Conclusion
AWS SQS is an excellent tool for managing message queues in distributed, decoupled applications. It provides reliable message delivery and scalable communication between services, making it ideal for microservices, serverless applications, and other distributed systems. By using the boto3
library in Python, you can easily interact with SQS, send and receive messages, and manage your queues programmatically.
In this tutorial, we covered the basics of SQS, its benefits, how to create a queue, send a message, and process messages with Python. Whether you're building a small application or a large-scale system, SQS can help ensure that your services communicate reliably and efficiently.