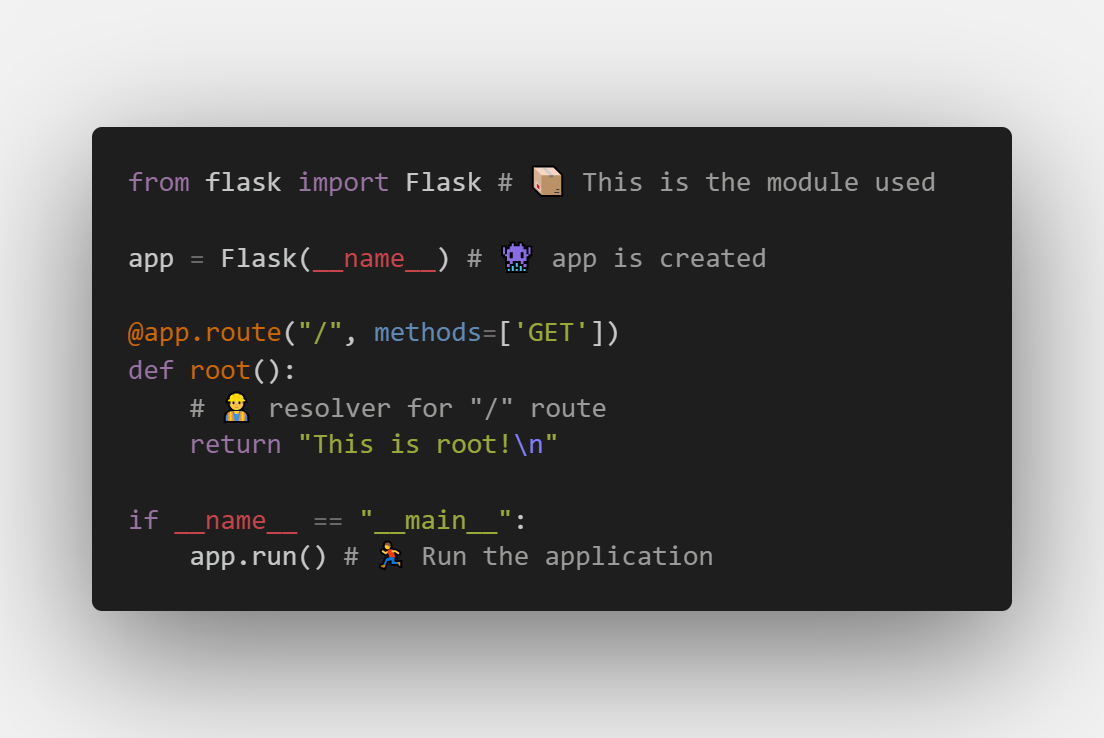
How API Endpoints Work in Python
When a client (e.g., a web browser or mobile app) wants to interact with a backend service, it sends a request to a server's API endpoint. The server processes the request and sends back a response, which the client can then use. In this example, we will explain how this process works using a simple API built with Python's Flask framework.
How a Client Sends a Request to an Endpoint
1. The client sends an HTTP request to a specific API endpoint. This request can use different HTTP methods such as GET, POST, PUT, or DELETE, depending on the action the client wants to perform.
2. In this example, the client sends a GET request to the endpoint /api/hello:
GET /api/hello HTTP/1.1
Host: 127.0.0.1:5000
How the Server Processes the Request
Once the server receives the request, it looks up the corresponding route (endpoint) and processes it. Here's how it works with the Flask app:
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/api/hello', methods=['GET'])
def hello_world():
return jsonify(message="Hello, World!")
if __name__ == '__main__':
app.run(debug=True)
In this example, Flask is used to define the route /api/hello
, which accepts GET requests. The function hello_world()
is executed when this endpoint is called. The function prepares a response and sends it back to the client.
Server Responds with Data
After processing the request, the server sends back an HTTP response. The response includes:
- Status Code: Indicates whether the request was successful (e.g., 200 OK).
- Response Body: Contains the data returned from the server, usually in JSON format.
- Headers: Metadata about the response (e.g., content type).
In our example, the server sends a response like this:
HTTP/1.1 200 OK
Content-Type: application/json
{
"message": "Hello, World!"
}
Client Receives and Handles the Response
The client receives the response from the server and can use the data. For example, a web browser will display the message to the user, or a mobile app might process the message further.
Steps of the Request-Response Process
- The client sends an HTTP request to a specific URL (the API endpoint).
- The server processes the request by matching the route and executing the corresponding function.
- The server responds with an HTTP response, including data (e.g., JSON) and a status code.
- The client handles the response and uses the data as needed.
Test the API Endpoint
To test this endpoint locally:
- Install Flask with the command:
pip install flask
python app.py
Benefits of Using APIs
- Modularity: APIs allow you to separate the frontend and backend, making it easier to manage and scale your application.
- Reusability: Once an API is built, it can be reused by different clients, including web browsers, mobile apps, or other services.
- Flexibility: You can easily update or change the backend logic without affecting the client applications.
- Integration: APIs can integrate with third-party services, allowing your application to access external data or services.
Considerations for Building APIs
- Ensure that your API is secure, especially if it handles sensitive data (e.g., through authentication and authorization).
- Make your API efficient and fast by optimizing the server's performance and handling requests properly.
- Consider using pagination, filtering, and caching to handle large datasets and improve the response times.
In conclusion, APIs are a powerful way to allow different applications to communicate. In this example, we showed how a simple Flask-based API endpoint works, from the client sending a request to the server processing it and sending back a response.